Sometimes it can be helpful to deprecate a field in the Schema without removing it altogether.
This snippet shows how to deprecate the `Post.excerpt` field.
You can use this technique to deprecate other fields.
// Filter the Object Fields
add_filter( 'graphql_object_fields', function( $fields, $type_name, $wp_object_type, $type_registry ) {
// If the Object Type is not the "Post" Type
// return the fields unaltered
if ( 'Post' !== $type_name ) {
return $fields;
}
// If the excerpt field doesn't exist
// (removed by another plugin, for example)
// return the fields unaltered
if ( ! isset( $fields['excerpt'] ) ) {
return $fields;
}
// Add a deprecation reason to the excerpt field
$fields['excerpt']['deprecationReason'] = __( 'Just showing how to deprecate an existing field', 'your-textdomain' );
// return the modified
return $fields;
}, 10, 4 );
After using this snippet, we can verify in the WPGraphQL Schema Docs that the field is indeed deprecated:
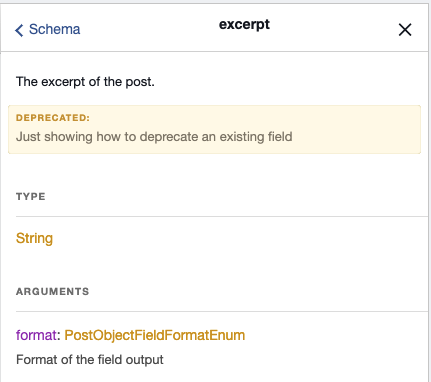