Add an Object Type to the GraphQL Schema
register_graphql_object_type( string $type_name, array $config );
Parameters
- $type_name (string): The name of the Type. This must be unique across all Types of any kind in the GraphQL Schema.
- $config (array):
- $description (string): Description of the Object Type. What does it represent? This will be used in Introspection for for self-documenting the Type in the Schema.
- $fields (array): Associative array of key/value pairs. The key being the field name and the value being the field config.
- $name (string): The name of the field
- $config (array): Config for the field to add to the Type
- $type: (string): The name of the GraphQL Type the field should return.
- $description (string): Description of the field. What does the field represent? This will be used in Introspection to self-document the Schema.
- $resolve (function): The function that will execute when the field is asked for in a query.
Source
File: access-functions.php
Examples
Below are some examples of using the function to extend the GraphQL Schema.
Register a new Type with a single field
This example shows how to register a new type called MyNewType
which contains a String
field.
add_action( 'graphql_register_types', function() {
register_graphql_object_type( 'MyNewType', [
'description' => __( 'Describe the Type and what it represents', 'replace-me' ),
'fields' => [
'testField' => [
'type' => 'String',
'description' => __( 'Describe what this field should be used for', 'replace-me' ),
],
],
] );
} );
You can view this type in the documentation section of GraphiQL:
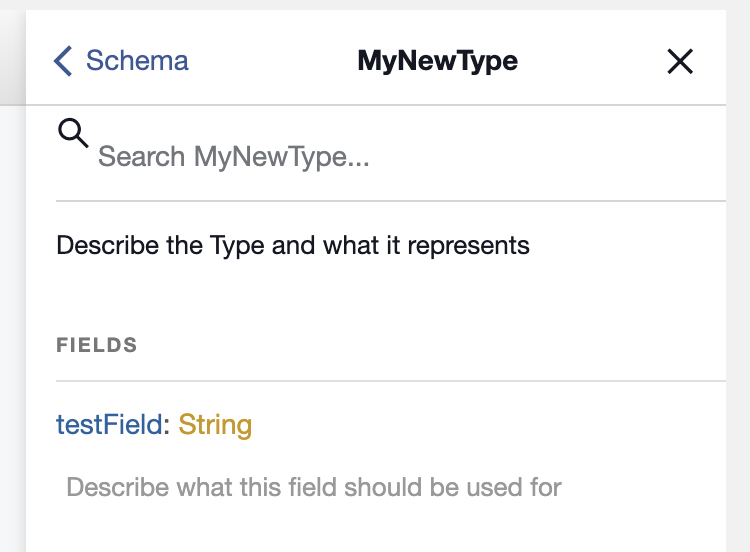
Register a new type with multiple fields
This example shows how to register a new type called MyMultifieldType
which contains a String
and an Int
field.
register_graphql_object_type( 'MyMultifieldType', [
'description' => __( 'Describe what MyMultifieldType is', 'replace-me' ),
'fields' => [
'testField' => [
'type' => 'String',
'description' => __( 'Describe what testField should be used for', 'replace-me' ),
],
'count' => [
'type' => 'Int',
'description' => __( 'Describe what the count field should be used for', 'replace-me' ),
],
],
] );
You can view this type in the documentation section of GraphiQL:
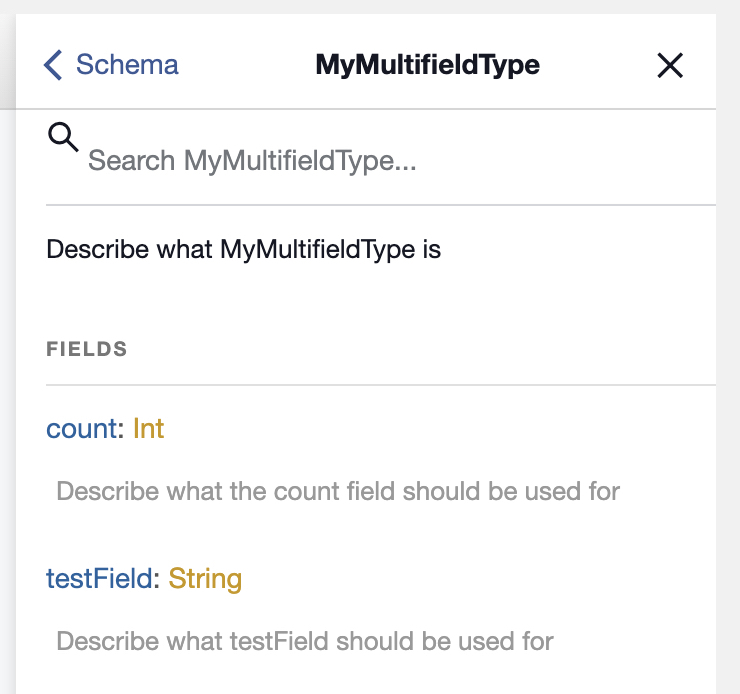