Given a Type Name and a $config array, this adds an EnumType to the TypeRegistry
register_graphql_enum_type( string $type_name, array $config );
Parameters
- $type_name (string): The unique name of the EnumType
- $config (array): Configuration for the EnumType
- $description (string): Description of the enum type. This will be used to self-document the schema and should describe to clients how the enum type should be used.
- $values (array): The possible values of the enum.
Source
File: access-functions.php
Examples
In GraphQL, Enum Types are used to provide a predefined set of values.
An example of an Enum in WPGraphQL is the AvatarRatingEnum
. Avatars in GraphQL can have a rating that is one of a predefined list of values: G
, PG
, R
, and X
. Since we know all the options before hand, we can expose fields to resolve to the AvatarRatingEnum
type and ensure that it’s always one of those predefined values.
Let’s say we wanted to be able to query for the current weather, and the responses should always be one of the following: Sunny
, Cloudy
, Rainy
register_graphql_enum_type( 'WeatherEnum', [
'description' => __( 'Condition of weather', 'your-textdomain' ),
'values' => [
'SUNNY' => [
'value' => 'sunny'
],
'CLOUDY' => [
'value' => 'cloudy'
],
'RAINY' => [
'value' => 'rainy'
],
],
] );
This would add an Enum to our Type registry, but it wouldn’t be in use yet. We could add a field that resolves to this Type:
register_graphql_field( 'RootQuery', 'currentWeather', [
'type' => 'WeatherEnum',
'description' => __( 'Get the weather', 'your-textdomain' ),
'resolve' => function() {
// Here you could fetch data from a database, an external API, or whatever you like.
// In this case, we'll just return static data. It has to return one of the values
// defined by the enum to fulfill the contract of the Schema.
return 'rainy'; //sunny, cloudy
},
] );
Now we could query:
{
currentWeather
}
And get a response like:
{
"data": {
"currentWeather": "RAINY"
}
}
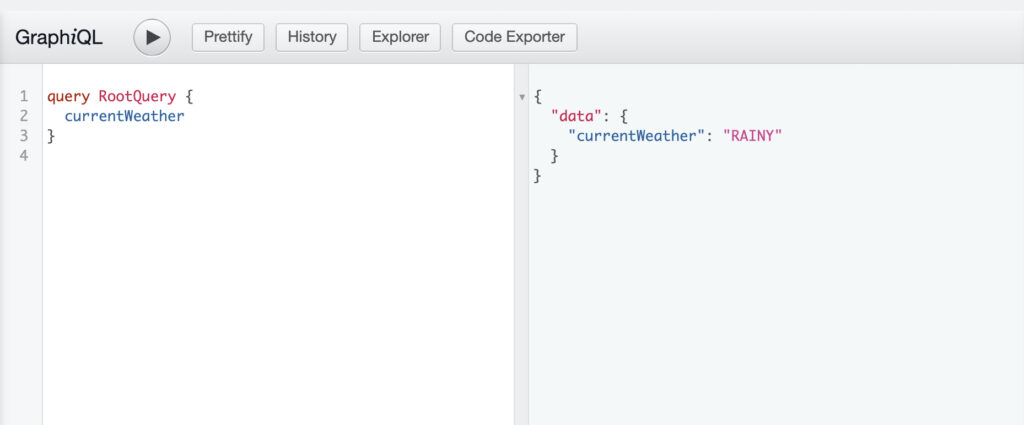