Given a config array for a custom Scalar, this registers a Scalar for use in the Schema
register_graphql_scalar( string $type_name, array $config );
Per the GraphQL Spec, WPGraphQL provides several built-in scalar types.
- Boolean
- Float
- Integer
- ID
- String
This function is used to define new Scalars to the Schema. Some common cases are Url, Email, Date, and others.
Note: Future versions of WPGraphQL will likely support some custom Scalars out of the box. Stay tuned!
Parameters
- $type_name (string): The name of the type to register
- $config (array): Configuration for the field
- $description (string): Description of the scalar. This will be used to self-document the schema and should describe how the scalar type should be used.
- $serialize (function): A function used to serialize the value of the scalar. Serializes an internal value to include in a response.
- $parseValue (function): A function used to parse the value of the scalar. Parses an externally provided value (query variable) to use as an input.
- $parseLiteral (function): A function used to parse the value of the scalar. Parses an externally provided literal value (hardcoded in GraphQL query) to use as an input.
Source
File: access-functions.php
Example
WPGraphQL ships with the out-of-the-box Scalars that are supported with GraphQL, however, it’s possible to define your own custom Scalars.
In order to register your own Scalar, you should understand how scalars are presented in GraphQL. GraphQL deals with scalars in following cases:
- When converting internal representation of value returned by your app (e.g. stored in a database or hardcoded in the source code) to serialized representation included in the response.
- When converting input value passed by a client in variables along with GraphQL query to internal representation of your app.
- When converting input literal value hardcoded in GraphQL query (e.g. field argument value) to the internal representation of your app.
In this example, we register a scalar called Email
.
register_graphql_scalar( 'Email', [
'description' => __( 'Email according to the email spec', 'wp-graphql' ),
/**
* Serializes an internal value to include in a response.
*
* @param string $value
* @return string
*/
'serialize' => function( $value ) {
return $value;
},
/**
* Parses an externally provided value (query variable) to use as an input
*
* @param mixed $value
* @return mixed
*/
'parseValue' => function( $value ) {
if ( ! filter_var( $value, FILTER_VALIDATE_EMAIL ) ) {
throw new Error( "Cannot represent following value as email: " . GraphQLUtilsUtils::printSafeJson( $value ) );
}
return $value;
},
/**
* Parses an externally provided literal value (hardcoded in GraphQL query) to use as an input.
*
* E.g.
* {
* user(email: "user@example.com")
* }
*
* @param GraphQLLanguageASTNode $valueNode
* @param array|null $variables
* @return string
* @throws Error
*/
'parseLiteral' => function( $valueNode, array $variables = null ) {
// Note: throwing GraphQLErrorError vs UnexpectedValueException to benefit from GraphQL
// error location in query:
if ( ! $valueNode instanceof GraphQLLanguageASTStringValueNode ) {
throw new Error( 'Query error: Can only parse strings got: ' . $valueNode->kind, [$valueNode] );
}
if ( ! filter_var($valueNode->value, FILTER_VALIDATE_EMAIL ) ) {
throw new Error( "Not a valid email", [ $valueNode ] );
}
return $valueNode->value;
}
]);
In order to view this new Scalar type, a new field can be registered.
register_graphql_field( 'RootQuery', 'testEmail', [
'type' => 'Email',
'description' => __( 'Testing custom scalars', 'wp-graphql' ),
'resolve' => function() {
return 'testemail@mac.com';
},
]);
This new field can now be queried:
{
testEmail
}
Which will result in the following data to be returned:
{
"data": {
"testEmail": "testemail@mac.com"
}
}
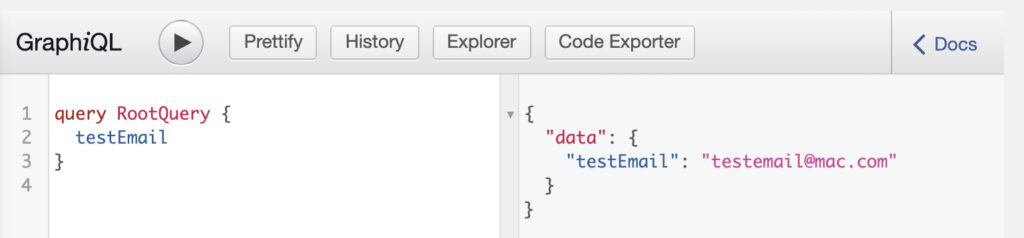
This new field and Scalar type can be viewed in the GraphiQL documentation:
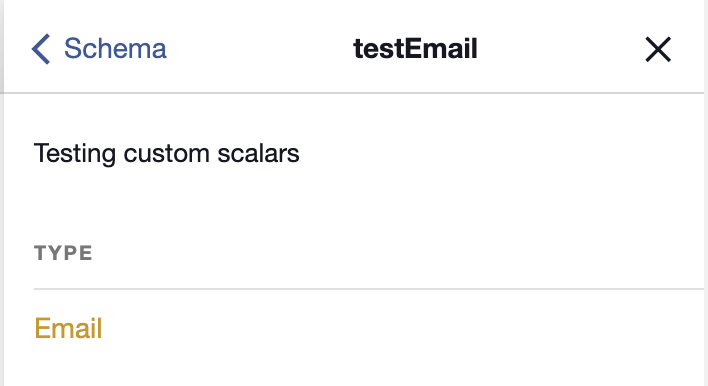