One of the most common ways to customize the WPGraphQL Schema is to register new fields.
When registering fields, argument(s) can also be defined for the field.
Field arguments in GraphQL allow input to be passed to the field, and when the field is resolved, the input of the field argument can be used to change how the field is resolved.
Registering Fields
Below is an example of registering a field with an argument:
add_action( 'graphql_register_types', function() {
$field_config = [
'type' => 'String',
'args' => [
'myArg' => [
'type' => 'String',
],
],
'resolve' => function( $source, $args, $context, $info ) {
if ( isset( $args['myArg'] ) ) {
return 'The value of myArg is: ' . $args['myArg'];
}
return 'test';
},
];
register_graphql_field( 'RootQuery', 'myNewField', $field_config);
});
Let’s break down the code:
Hooking into WPGraphQL
add_action( 'graphql_register_types', function() { ... });
This action hooks into WPGraphQL when the WPGraphQL Schema is being generated. By hooking our code here, it makes sure our function is only executed when WPGraphQL is being used.
Define the Field Config
Within that action, we define a $field_config
array which gets passed to the register_graphql_field()
function.
$field_config = [
'type' => 'String',
'args' => [
'myArg' => [
'type' => 'String',
],
],
'resolve' => function( $source, $args, $context, $info ) {
if ( isset( $args['myArg'] ) ) {
return 'The value of myArg is: ' . $args['myArg'];
}
return 'test';
},
];
Within the field config we define the following:
- type: We define the type as “String” to tell WPGraphQL that the field is a String in the Schema
- args: We define an array of arguments that will be available to the field.
- resolve: We define a function to execute when the field is queried in GraphQL. Resolve functions in GraphQL always receive 4 arguments ($source, $args, $context, $info). The argument we care about for this example is the 2nd one, $args. We check to see if that argument is set, and if it is, we append the value to the string “The value of myArg is:” and return it. Otherwise we just return the string “test”.
Register the Field
And now we can register the field using the $field_config we have defined:
register_graphql_field( 'RootQuery', 'myNewField', $field_config);
Here we use the register_graphql_field()
function. It accepts 3 arguments:
- The first argument is the Type in the Schema to add a field to. In this case, we want to add a field to the RootQuery Type.
- The second argument is the name of the field we are registering. This should be unique on the Type, meaning the Type should not already have a field of this name.
- The third argument is the $field_config, which we just reviewed.
The field in action
We can query this like so:
query {
myNewField
}
and the results will be:
{
"data": {
"myNewField": "test"
}
}
Now, we can pass a value to the argument like so:
query {
myNewField( myArg: "something" )
}
and the results will be:
{
"data": {
"myNewField": "The value of myArg is: something"
}
}
Now, you can introduce GraphQL variables like so:
query MyQuery($myArg:String) {
myNewField( myArg: $myArg )
}
And then you can pass variables to the request. Here’s an example of using a variable in GraphiQL:
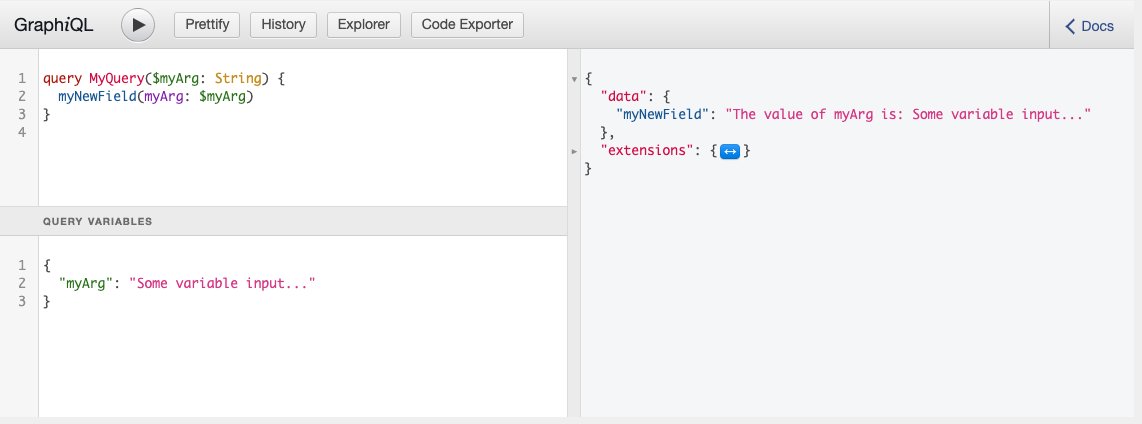