Given a Type Name and a $config array, this adds an Interface Type to the TypeRegistry
register_graphql_interface_type( string $type_name, array $config );
Parameters
- $type_name (string): The unique name of the Interface Type
- $config (array): Configuration for the field
- $description (string): Description of the interface type.
- $fields (array): The fields that are part of the interface type.
- $type (string): The field type
- $description (string): The field description
- $resolveType (function): A function that takes the resolving data and determines what GraphQL Type should be returned
Source
File: access-functions.php
Examples
Below are some examples of using the function to add Interface types to the GraphQL Schema.
Register a custom interface type
This example adds an Interface Type
called MyInterface
to the GraphQL Schema with a test field called myTestField
.
register_graphql_interface_type( 'MyInterface', [
'fields' => [
'myTestField' => [
'type' => 'String',
'resolve' => function() {
return 'myTestField is working';
},
],
],
]);
The above snippet registers the Interface to the Schema, but it’s not used anywhere, yet.
Using the Interface
In order to use this interface, it needs to be registered to an Object Type in the Schema.
This can be done by registering a new object type that implements the interface, or by registering the interface to an existing object type.
In this example, we use the register_graphql_interfaces_to_types
function to apply the interface to the existing Post
type.
register_graphql_interfaces_to_types( ['MyInterface'], ['Post'] );
You can now query the interface:
{
posts {
nodes {
myTestField
id
}
}
}
And get results containing the value resolved for myTestField
{
"data": {
"posts": {
"nodes": [
{
"myTestField": "myTestField is working",
"id": "cG9zdDo1"
},
{
"myTestField": "myTestField is working",
"id": "cG9zdDox"
}
]
}
}
}
Here is how this looks inside GraphiQL:
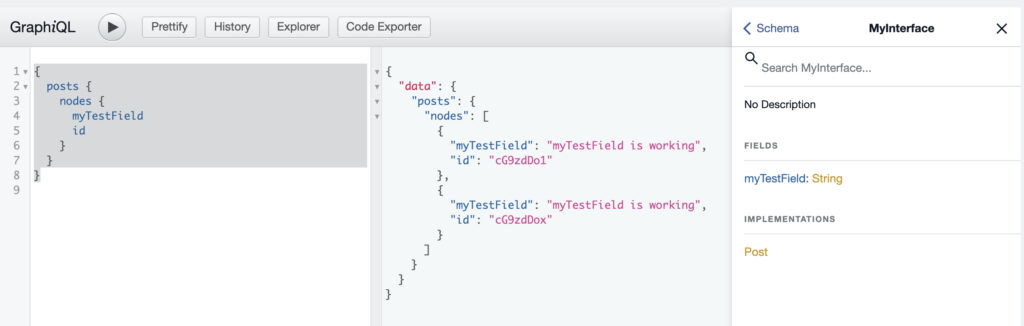